Streaming Order Book - Project
A mini project written in Rust that connects to two exchanges' WebSocket feeds simultaneously, pulls order books for a given traded pair of currencies from each exchange, merges and sorts the order books to create a combined order book, and publishes the spread, top ten bids, and top ten asks as a stream through a gRPC server.
Websocket Test Page (Order Book Visual)
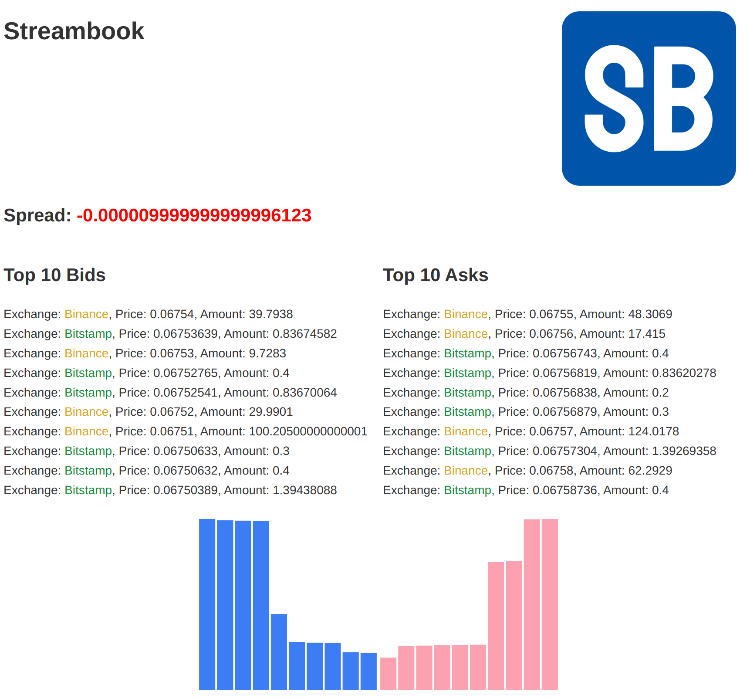
Requirements
Rust (version 1.5 or later)
Installation
-
Clone the repository:
git clone https://github.com/mbarth/stream-book.git
-
Change to the project directory:
cd stream-book
-
Build the project:
cargo build --release
Usage
-
The
local.toml
file under the/config
directory holds the current URLs for the two exchanges. Make any changes necessary if you would like a different currency pair. Currently, the currency pair used isETHBTC
.[exchanges.binance] address = "wss://stream.binance.com:9443/ws/ethbtc@depth20@100ms" [exchanges.bitstamp] address = "wss://ws.bitstamp.net" event = "bts:subscribe" channel = "order_book_ethbtc"
-
Start the gRPC server:
cargo run --release
-
The gRPC server will be running and ready to accept client connections. You can now connect to the server and consume the streaming spread, top ten bids, and top ten asks. The grpcurl utility is an easy way to connect and test the gRPC server endpoints.
# using reflection grpcurl -d '{}' -plaintext localhost:50051 orderbook.OrderbookAggregator/BookSummary # under the project's root directory and using the protobuf file grpcurl -plaintext -import-path ./proto -proto orderbook.proto -d '{}' 'localhost:50051' orderbook.OrderbookAggregator/BookSummary
-
Alternatively, there is a websocket endpoint available that returns similar data. The websocat utility is a simple client to view the results from the websocket endpoint.
websocat ws://localhost:8080/ws
-
Lastly, there is a webpage built using the websocket endpoint available at http://localhost:8080 that can be used to visualize the results.
-
To stop the server, issue a
ctrl+c
command in the same terminal where the service was started.
Testing Instructions
-
To run the unit tests:
cargo test
Configurable Options
- The number of Top Bids and Asks displayed is controlled by the
app.top_bids_and_asks_count
option. By default, the Bitstamp exchange returns 100 results and Binance results is controlled by itsdepth20
value, here returning 20 results. Therefore, at most this value can be set to 120. Set this value accordingly to your results setting.
Credit
-
Thanks to @idibidiart for a sample of how to render the bids/asks chart using D3.js. The code is modified to work with streaming websocket data.
-
Thanks to @dgtony for their OrderBook Bid/Ask ordering logic, lines 18-53. It's modified slightly but the logic is basically the same.